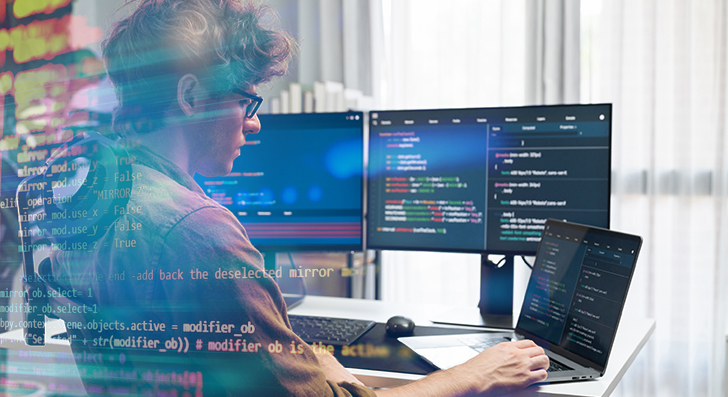
Scalability indicates your software can take care of development—more buyers, far more info, and much more traffic—without breaking. For a developer, creating with scalability in your mind will save time and strain later. Below’s a clear and sensible guideline to assist you to start out by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability is just not anything you bolt on later on—it ought to be aspect of your approach from the beginning. Many purposes are unsuccessful every time they mature rapidly due to the fact the initial design and style can’t handle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Commence by building your architecture to become flexible. Prevent monolithic codebases where almost everything is tightly connected. In its place, use modular style or microservices. These styles split your app into scaled-down, independent elements. Just about every module or service can scale on its own devoid of influencing the whole program.
Also, think about your database from day just one. Will it need to handle 1,000,000 end users or merely 100? Pick the appropriate form—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential place is to stay away from hardcoding assumptions. Don’t write code that only functions below present situations. Think of what would come about If the consumer foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use layout designs that help scaling, like message queues or occasion-driven systems. These aid your app deal with much more requests with out obtaining overloaded.
Whenever you build with scalability in your mind, you are not just planning for achievement—you happen to be minimizing potential head aches. A well-prepared process is simpler to maintain, adapt, and grow. It’s improved to prepare early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is really a vital Component of constructing scalable programs. Not all databases are developed the same, and utilizing the Improper one can sluggish you down or perhaps cause failures as your application grows.
Begin by understanding your facts. Can it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. They also guidance scaling strategies like browse replicas, indexing, and partitioning to deal with more targeted traffic and information.
If the information is much more flexible—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more very easily.
Also, look at your study and publish styles. Have you been executing plenty of reads with less writes? Use caching and skim replicas. Are you handling a heavy compose load? Check into databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your accessibility patterns. And usually check database effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s construction, speed requirements, and how you anticipate it to increase. Just take time to choose wisely—it’ll save a lot of trouble afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact delay provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting clean up, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions brief, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code requires much too prolonged to run or employs an excessive amount of memory.
Subsequent, evaluate your database queries. These normally slow matters down over the code alone. Ensure each query only asks for the info you actually will need. Keep away from SELECT *, which fetches almost everything, and instead decide on specific fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across massive tables.
If you recognize exactly the same knowledge remaining requested over and over, use caching. Retail store the results briefly working with tools like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 data could possibly crash when they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and even more targeted traffic. If almost everything goes by way of one particular server, it is going to speedily turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming website traffic throughout a number of servers. As opposed to a single server performing every one of the perform, the load balancer routes customers to different servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to create.
Caching is about storing info quickly so it could be reused swiftly. When users ask for the identical information yet again—like here a product web site or maybe a profile—you don’t must fetch it from the databases each time. You are able to provide it from your cache.
There's two widespread types of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for fast entry.
2. Customer-facet caching (like browser caching or CDN caching) retailers static data files near the consumer.
Caching cuts down database load, increases speed, and can make your app a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when information does adjust.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application tackle much more people, stay quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Applications
To construct scalable programs, you require tools that let your app expand quickly. That’s where cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get hardware or guess foreseeable future ability. When website traffic improves, you could increase extra resources with just some clicks or automatically using auto-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety resources. You are able to focus on building your application in lieu of taking care of infrastructure.
Containers are One more essential Instrument. A container packages your application and anything it should operate—code, libraries, options—into a single unit. This can make it effortless to move your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container resources indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to expand devoid of limits, start out utilizing these equipment early. They help you save time, minimize possibility, and assist you to keep centered on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of creating scalable devices.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently glitches transpire, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes higher than a Restrict or maybe a assistance goes down, it is best to get notified promptly. This will help you resolve concerns quick, frequently before buyers even detect.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you keep in control.
Briefly, monitoring can help you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works well, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the correct instruments, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start modest, Imagine large, and Create smart.